Difficulty: Easy
Skills Used: following the mouse, Layer Masks, Event Listeners, registration points
This is a simple movie to make, but it can be used for lots of applications. A larger version of the map sits in a layer on top of the original. A layer mask hides the large map except for within the circle of the magnifying glass. The large map also moves back and forth according the movement of the mouse.
Instructions:
1. Find a large picture to use for your tutorial. (Remember, always start with a big picture and then make it smaller, don't start with a small picture and make it bigger.) It doesn't have to be a map, but for this tutorial we will assume it is. You can download the map of Riverside at the end of this tutorial if you would like.
2. Open the large map in Photoshop and create a smaller version. My magnifying glass magnifies things by 2.5, so to make my smaller picture, I divided the width and height of the large map by 2.5.
Original Map Divide By Small Map
Width: 936 px /2.5 = 374 px
Height: 1071 px /2.5 = 428 px
Don't know how to use Photoshop? You can learn it in just two hours! Click here to download the tutorial.
3. Open a new file in Flash. Make sure you choose Actionscript 3.0. Right click on the grey area around the stage and choose Document Properties from the menu that pops up. Set the stage width and height to that of your small picture, in my case 374 x 428.
4. Import your large and small map to the library. Create a layer in the timeline and call it "Small Map". Place the small map in that layer and center it so it covers the entire stage. Create a second layer and call it "Big Map". Drag the large map into that layer. (It does not have to be centered on the stage, as it will be positioned through AS3. You can put it to the side to make it easier to see the small map.)
5. In the Properties Inspector name the instance of your large map "myMap". Create a third layer in the timeline and name it "Actionscript". Open up the Actions window and add this code:
myMap.addEventListener(Event.ENTER_FRAME, magnify);
function magnify(e:Event)
{
myMap.x = (mouseX * -1.5);
myMap.y = (mouseY * -1.5);
}
The event listener means the movie will read this code every time it enters a new frame. The large map is 2.5 times as big as the small map, so for every one unit the mouse moves, the large map moves another 1.5 units. 1 + 1.5 = 2.5. Get it? Test your movie and make sure the large map is moving around properly.
6. Create another layer above "Big Map" and name it Glass. Use the oval tool to create a circle in this layer. Mine is 100 x 100. It doesn't matter what color the fill is, you won't be able to see it. However, you will see the color of the stroke. Mine is 996600. Hold down the shift key while you are creating the shape so you get a circle and not an oval.
7. Select the stroke around the circle, then choose Edit -> Cut. We will be pasting the rim of the circle in a separate layer.
8. Right click the circle and select "Convert to Symbol" from the pop up menu. Convert it to a movie clip, and name it "myCircle" or "magnifying glass", something you will recognize. In the properties inspector, name this instance "myCircle".
9. In the library, double click on the magnifying glass. Use the align tools (align horizontal center, align vertical center) to center the registration point. If you don't do this, the magnifying glass will be just below and to the right of the mouse.
10. Open up the Actions window again and add the following code to the function "magnify" that we created before.
myCircle.x = mouseX;
myCircle.y = mouseY;
Now the circle will always follow the mouse. Test your movie to make sure the circle is moving around properly.
11. Double check that the layer "Glass" is right above the layer "Big Map". Right click on the "Glass" layer and select "Mask" from the pop up menu. "Big Map" should now be under "Glass" and slightly indented. You have created a layer mask. Now you will only be able to see part of the big map that lies within the circle.
12. Create a layer above "Glass" called "Rim". Paste the stroke of the circle we cut earlier into the "Rim" layer. Right click the rim and convert it to a symbol. Call it "Rim" or whatever you'd like. In the properties inspector, name this instance of the rim "myRim". In the library, double click on the rim and use the align menu to center it over its registration point.
13. In the actions window, add the following code to the "magnify" event.
myRim.x = mouseX;
myRim.y = mouseY;
Test your movie and make sure the rim is centered over the glass and following your mouse.
14. Last step! You can still see the mouse in the center of the magnifying glass, and of course we don't want that. In the actions window, add the following code (I like to keep this at the top.)
import flash.events.Event;
import flash.events.MouseEvent;
import flash.ui.Mouse;
(This imports the information Flash needs to show and hide your mouse. If you don't add it, you might think your movie is working fine, but if you upload it to a website your mouse will be visible again.) Then add these two functions at the bottom of your code.
stage.addEventListener(Event.MOUSE_LEAVE, showMouse);
function showMouse(evt:Event):void
{
Mouse.show();
}
stage.addEventListener(MouseEvent.MOUSE_MOVE, hideMouse);
function hideMouse(evt:Event):void
{
Mouse.hide();
}
The first function allows the mouse to show whenever it leaves the stage. The second function hides the mouse whenever it moves within your movie.
You're done! Test your movie and make sure it works.
Final Code:
import flash.events.Event;
import flash.events.MouseEvent;
import flash.ui.Mouse; /*don't forget this part, otherwise the mouse will show if you post this to a website
*/
myMap.addEventListener(Event.ENTER_FRAME, moveBigMap);
function moveBigMap(e:Event)
{
/*the big map is 2.5 times bigger than the small map
for every one unit the mouse moves, the big map movies 1.5 units, 1 + 1.5 = 2.5*/
myMap.x = (mouseX * -1.5);
myMap.y = (mouseY * -1.5);
/*move the magnifying glass*/
myCircle.x = mouseX;
myCircle.y = mouseY;
/*move the rim of the magnifying glass*/
myRim.x = mouseX;
myRim.y = mouseY;
}
/*if the mouse leaves the map, show the mouse*/
stage.addEventListener(Event.MOUSE_LEAVE, showMouse);
function showMouse(evt:Event):void
{
Mouse.show();
}
/*if the mouse moves anywhere on the stage, show the mouse*/
stage.addEventListener(MouseEvent.MOUSE_MOVE, hideMouse);
function hideMouse(evt:Event):void
{
Mouse.hide();
}
Download the Image Files
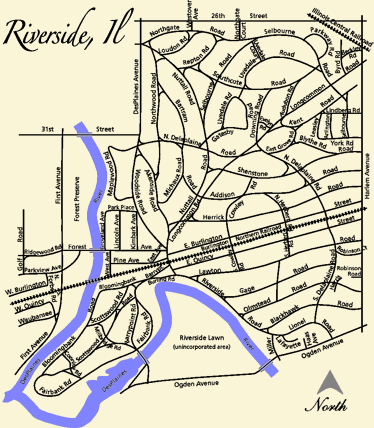
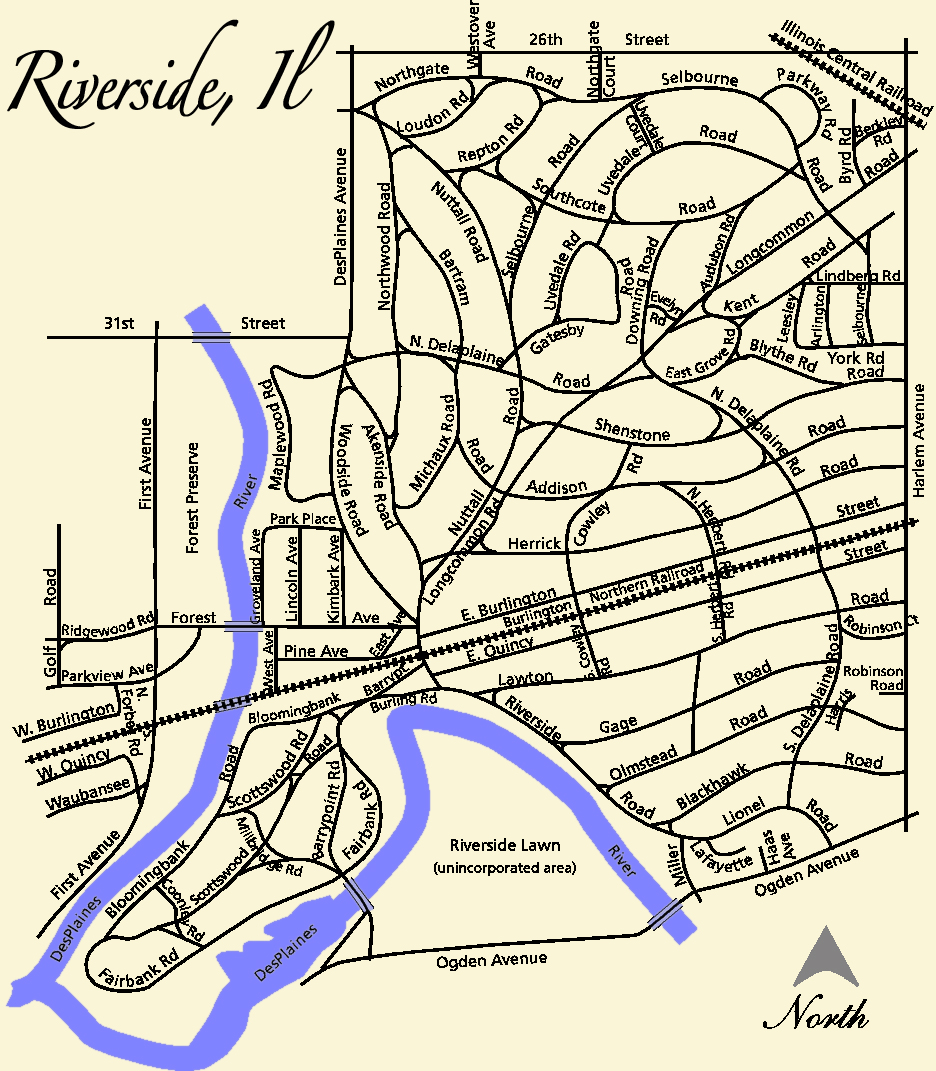
No comments:
Post a Comment