Difficulty: Easy
Skills Used: addChild, removeChild, depth, sound effects, arrays, for loops, event listeners, registration points, particles, key events
Make a working Etch a Sketch. Draw anything your little heart desires using your arrow keys, then erase it by hitting "Erase!"
Instructions:
1. Make a new file in Flash. Make sure it's Actionscript 3.0. Right click on the grey area next to the stage and select "Document Properties". Set the stage to 480 x 360 pixels.
2. In the timeline, create five layers and name them (from top to bottom) "Actions", "Erase", "Knobs", "Frame", and "BG". Download the picture of the Etch a Sketch from the bottom of this tutorial, import it to your library, and place it in the "BG" layer. Center it so it covers the entire stage.
3. We want the Etch a Sketch "drawing" to appear to be under the red frame, not on top of it. Download the frame from the bottom of this tutorial (download it, don't just copy and paste or you'll have a white background) and import it to your library. Drag it into the "Frame" layer and center it so it is directly above the background picture.
4. Even though we're not really using the knobs on this Etch a Sketch, it's still fun to make them turn. Download the picture of the knob (download it, if you copy and paste you'll get a white background) and import it into your library. Drag the knob into your "Knob" layer and place it directly over the left knob on the Etch a Sketch frame. (X: 45, Y: 324.5) Right click the knob and select "Convert to Symbol". It doesn't matter what you name the movieclip, just call it "knob". But in the properties inspector, give the instance of the knob the name "leftKnob". I rotated my left knob 90 degrees clockwise to make it look a little different from the right knob.
5. Drag a second instance of your knob movieclip into the "Knobs" layer and place it directly over the right knob on the Etch a Sketch frame. (X: 442.5, Y: 325) In the properties inspector, name this second instance "rightKnob".
6. In the top menu, select Insert -> New Symbol. Name the symbol "particle".Use the Oval Tool to make a circle that is .75 x .75, with no stroke, and fill it with 555555. Use the align menu to center it over the registration point. (The registration point is that little cross in the center of the screen.) Go to the library. Find the particle movie clip. Double click to the right of "particle", under the "Linkage" column, and type in "particle". So now it should say "particle" under "Name" and under "Linkage".
7. Go back to the mainframe of the movie by clicking "Scene 1" in the upper left corner. Select the "Actions" layer in the timeline. Open the Actions window and paste in the following code...
import flash.display.Sprite;
import flash.events.KeyboardEvent;/*Important! Otherwise when you post your swf to the web the keys won't register.*/
var Xloc:uint = 63;/*where the particles will be drawn on the stage*/
var Yloc:uint = 61;/*where the particles will be drawn on the stage*/
var particleArray:Array = new Array();/*an array to keep the particles organized*/
stage.addEventListener(KeyboardEvent.KEY_DOWN, sketch);/*when the user presses a key, call the sketch function*/
function sketch(event:KeyboardEvent):void
{
switch (event.keyCode)
{
case 37:
//left
if (Xloc > 58)
{
Xloc -= 1;//adjust the left/right position
leftKnob.rotation -= 5;/*rotate the knob*/
}
break;
case 39:
//right
if (Xloc < 425)
{
Xloc += 1;
leftKnob.rotation += 5;
}
break;
case 38:
//up
if (Yloc > 56)
{
Yloc -= 1;
rightKnob.rotation -= 5;
}
break;
case 40:
//down
if (Yloc < 299)
{
Yloc += 1;
rightKnob.rotation += 5;
}
break;
default:
trace (event.keyCode)/*this is a good way to look up the keycode for a key you don't know*/
}
/*draw a particle on the screen*/
var particleMC = new particle();
particleMC.x = Xloc;
particleMC.y = Yloc;
addChildAt(particleMC,1);/*we're drawing the particles at depth 1, which is above the background but below everything else*/
particleArray.push(particleMC);/*adds drawn particles to an array, will make it easy to erase them later*/
}
This creates an event listener that waits for the user to press a key. Every time the user presses an arrow key, it moves the location where a particle will be drawn either left, right, up, or down. Then it draws the particle, and adds it to an array to keep things organized. Notice that I'm using "addChildAt" instead of "addChild". This draws the particles at depth 1, just above the background layer, so it won't appear on top of the frame.
Test your movie. You should be able to draw on your Etch a Sketch using the arrow keys, and the knobs should turn.
8. The app is almost finished, but there's no way to erase your drawing. We need to fix that. Download the Erase button from the bottom of the tutorial. (Download it, don't just copy and paste.) Import it to your library, drag it to the "Erase" layer, and use the properties inspector to move it to X: 177, Y: 301. Right click the button and select "Convert to Symbol". Name the movieclip "erase", or whatever you want, doesn't really matter. Then in the properties inspector name this instance of the movieclip "erase".
9. (Optional) Find or record a sound effect for "shaking" the Etch a Sketch. I recorded myself gently shaking some rice in a plastic bag using Audacity. Import it to your library. Double click next to your mp3 file, under "Linkage" and name it "shake".
10. Select the "Actions" layer and open the actions window again. Add the following code to the bottom of the window:
erase.addEventListener(MouseEvent.CLICK, eraseParticles);/*when you click the erase button, it calls the eraseParticles function*/
var shakeEffect:Sound = new Sound();
shakeEffect = new shake();
var shakeChannel:SoundChannel = new SoundChannel();/*get the shake sound ready*/
function eraseParticles(event:MouseEvent):void
{
shakeChannel = shakeEffect.play();/*play shake sound effect*/
for (var i:uint=particleArray.length-1; i>0; i--)/*kind of backwards, but this way I can use the pop method*/
{
removeChild(particleArray[i]);/*removes particle from stage*/
particleArray.pop();/*removes last item from array*/
}
}
This puts an event listener on the erase button so when the user clicks it, it calls the eraseParticles function. The function plays the sound effect and uses a backwards forloop to erase each particle one by one and delete it from the array. If you're not using a sound effect, erase or comment out the four lines that contain the word "shake".
You're done! Test your movie and make sure it's drawing and erasing properly.
Final Code:
import flash.display.Sprite;
import flash.events.KeyboardEvent;/*Important! Otherwise when you post your swf to the web the keys won't register.*/
var Xloc:uint = 63;/*where the particles will be drawn on the stage*/
var Yloc:uint = 61;/*where the particles will be drawn on the stage*/
var particleArray:Array = new Array();/*an array to keep the particles organized*/
stage.addEventListener(KeyboardEvent.KEY_DOWN, sketch);/*when the user presses a key, call the sketch function*/
function sketch(event:KeyboardEvent):void
{
switch (event.keyCode)
{
case 37:
//left
if (Xloc > 58)
{
Xloc -= 1;//adjust the left/right position
leftKnob.rotation -= 5;/*rotate the knob*/
}
break;
case 39:
//right
if (Xloc < 425)
{
Xloc += 1;
leftKnob.rotation += 5;
}
break;
case 38:
//up
if (Yloc > 56)
{
Yloc -= 1;
rightKnob.rotation -= 5;
}
break;
case 40:
//down
if (Yloc < 299)
{
Yloc += 1;
rightKnob.rotation += 5;
}
break;
default:
trace (event.keyCode)/*this is a good way to look up the keycode for a key you don't know*/
}
/*draw a particle on the screen*/
var particleMC = new particle();
particleMC.x = Xloc;
particleMC.y = Yloc;
addChildAt(particleMC,1);/*we're drawing the particles at depth 1, which is above the background but below everything else*/
particleArray.push(particleMC);/*adds drawn particles to an array, will make it easy to erase them later*/
}
erase.addEventListener(MouseEvent.CLICK, eraseParticles);/*when you click the erase button, it calls the eraseParticles function*/
var shakeEffect:Sound = new Sound();
shakeEffect = new shake();
var shakeChannel:SoundChannel = new SoundChannel();/*get the shake sound ready*/
function eraseParticles(event:MouseEvent):void
{
shakeChannel = shakeEffect.play();/*play shake sound effect*/
for (var i:uint=particleArray.length-1; i>0; i--)/*kind of backwards, but this way I can use the pop method*/
{
removeChild(particleArray[i]);/*removes particle from stage*/
particleArray.pop();/*removes last item from array*/
}
}
Download the Image Files:
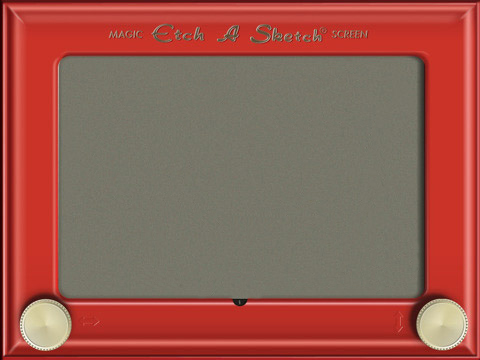
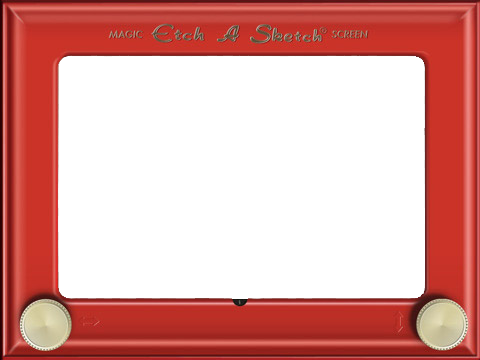

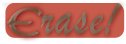
No comments:
Post a Comment